In modern times QR codes should be known by pretty much everyone. Since smartphones are becoming increasingly popular, QR codes can also be found at more and more places in our everyday lives. As a fairly serious geek this should be reason enough to engage a bit more in detail with the technology behind it. And what way seems to be more suitable than implementing a QR code generator by yourself?
Because C# is my favourite programming language, the choice fell accordingly to this language. Sadly information on the QR code are not so easy to get. The easiest way of course would be the official way – simply buy the ISO/IEC document. That would be the ISO/IEC 18004. But there’s also a crux on that. The ISO 18004 costs round about 232$, which is a little too expensive for a non-profit hobby project, however.
How does QR codes work (technically)?
Luckily, there are some really good sources on the internet, that explain the functional principles of a QR code generator. With the help of these sites, I got down to my own implementation. For those who want to read up on the technical side of the subject, I listed below my main sources for you:
Since the complete logic behind the QR code generation process is way more complicated and beyond the scope of this post, I will not explain this further here. You might want to read up on the pages linked above and if you have any questions, of course, you can write me a comment. I’ll then give my best to answer your questions.
I have already implemented several QR code solutions for customers in various industries. So why not for you?
In the following I like to show you how to use my library and under what license it is published.
From where can I get QRCoder?
You can get the source code of QRCoder, that’s how I called my QR code generator, on GitHub. The code is licensed under the MIT license, so that QRCoder can be used in non-commercial as well as commercial projects.
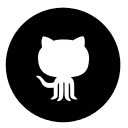
QRCoder @ Github
Nevertheless, if you use the library for one of your projects, I would be happy if you would leave a short comment. (It’s always nice to see what will become of his “code babies”.)
How to use QRCoder?
The usage of QRCoder is relatively simple. At first you must (of course) add a reference to the QRCoder.dll. To create a QR code and display it you need only 4 more lines of code.
private void renderQRCode() { QRCodeGenerator qrGenerator = new QRCodeGenerator(); QRCodeData qrCodeData = qrGenerator.CreateQrCode("The text which should be encoded.", QRCodeGenerator.ECCLevel.Q); QRCode qrCode = new QRCode(qrCodeData); Bitmap qrCodeImage = qrCode.GetGraphic(20); }
In the first line the QR code generator is instantiated, in the second line a QR code data object is created, in the third line the QR code object is created and in the fourth line, the QR code is rendered as a bitmap and displayed in a PictureBox. That’s it! There is nothing more to do, to create a QR code and show it.
The appropriate error correction level
The CreateQrCode() function takes two arguments. At first the string which shall be encoded in the QR code and as second argument the error correction level (ECCLevel). Here, the levels L (7%), M (15%), Q (25%) and H (30%) are available, whereby the percentage indicates how much of the QR-code can be hidden/destroyed until the error correction algorithm can’t recreate the original message which was encoded in the QR code.
Be creative – render the QR code your way!
As shown above, a QR code can be rendered as Bitmap by using the GetGraphic() function. When using this function a classic layouted, black and white QR code graphic is generated. If you are a fan of colorful and fancy graphics, you may want to render your QR code in a more beatiful way than the given solution by using the GetGraphic() function.
No problem! Therefore I added the ModuleMatrix property which gives you a list of BitArrays (which are representing logical lines). So, for example, if in list index 3 (line 2) on BitArray index 4 (column 3) the bool value is true, then you have to render this block element as dark element. If the value is false, it has to be rendered as light element.
Now, by iterating through the List<BitArray> ModuleMatrix, you can implement your on function to render the QR code.
//List<System.Collections.BitArray>ModuleMatrix foreach (System.Collections.BitArray line in qrCode.ModuleMatrix) { foreach (bool block in line) { //Write your own rendering algorithm here //When block==true then render dark, else render light } }
Feedback and feature plans
If you have (constructive) feedback, ideas, questions, tips or problems with the library, then just write me a comment. Also everytime somebody of you forks this project on GitHub I’ll be really happy.
Because I’m running a little bit out of time at the moment, I’m not able to give something like a clear and precise roadmap. Nevertheless there are some ideas I really like to implement in the future. For example some more custom rendering algorithms/functions. Also I can imagine some more functions to create QR codes for special purposes, like a function which takes WiFi credentials and renders them as QR code. Or a function which takes mobile numbers or vcard.vcf files and renders them or … This thing now exists. Try the PayloadGenerator class and generate your favourite payload.
But for all that I need time. So if you are motivated, talented and want to bring this QR code library to the top, fork it on Github and work together with me.
So far, so good. I hope you enjoyed reading this article and wish you a lot of fun with the QRCoder library!
This is such an informative post about QR code generation! The details you’ve provided are not only helpful but also inspiring for tech enthusiasts who want to dive into such projects. On another note, if you’re exploring entrepreneurial ventures, nurse practitioner business ideas are a growing trend in healthcare. Opportunities like telehealth platforms, wellness clinics, and mobile healthcare services are reshaping the industry and offer immense potential. Keep up the great work with these insightful posts!